Completing an introductional course on programming with Processing I wrote various little programms.
These so called sketches mostly feature space, sound, colors and patterns.
Assignment for the final codes
Timebased patterns:
Over the last 2 weeks you had a closer look into pattern observations.
The should be your inspiration for a programmed sketch. Start from your observations and your natural descriptions. Think about a pattern you want to create. The pattern can be based on input data (like an image or a text) or can be created from complexer shapes.
Transfer this pattern into a timebased pattern - an animation. Think about different behaviours of your elements. Use one function or several ones to build up your programme.
I tried to display some of them within the browser via Processing.js. Basically it works good enough for a quick demonstration, but the overall performance is really bad and it produces some other problems.
You can find some of the codes beneath the sketches' descriptions.
Of course you can download all programs already compiled in a package; they contain executable files and should work on every major operating system.
Soundvirus
The Soundvirus is a three dimensional sound visualization. It consists of four levels of branches, rising out of a common origin. Each branch has several children decreasing in quantity with higher branch levels. The whole system moves in time, while the 360 branches in total access 90 randomly generated variables based on their position in the field to calculate their movement in the three dimensions. This means that for most branches there are three other branches following the same movement, producing a mix of random arrangement and a subliminal pattern. The speed of the system's evolution, as well as its color are based on the stereo mix level of the sound input.
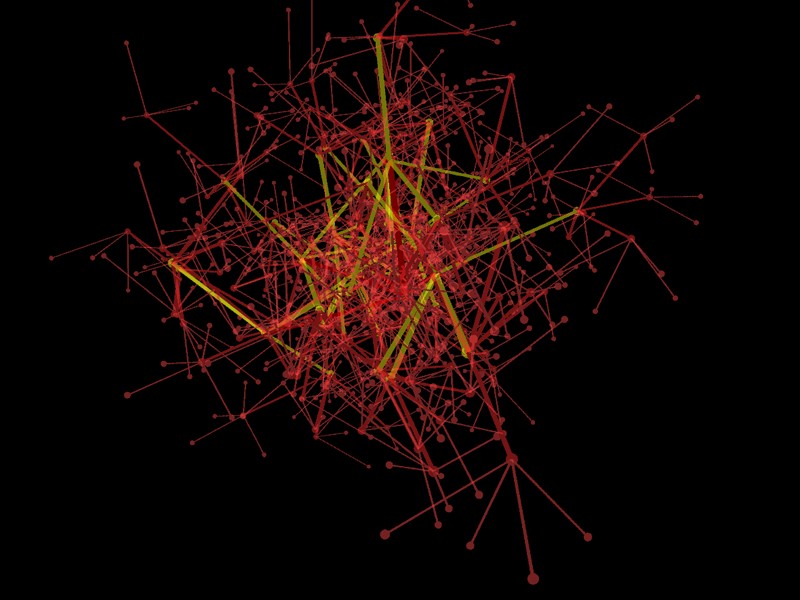
For the soundvirus_mic version, the microphone input is used. The microphone's sensivity can be changed by adjusting the "volumeadjust" variable in the first line. A smaller number increases the microphone's sensivity.
The soundvirus sketch uses the provided sound file to obtain the mix level.
Download Soundvirus
Download Soundvirus_mic
Dimension Clock
The Dimension Clock is a three dimensional way to display time. Hours, minutes and seconds are displayed as lines arranged in a circle on three different plains in the room. They are pointing away from the center and change in quantity and length as time goes by. The time is still shown in its numeric value to prevent confusion. By moving the mouse you can change the angle of view, a click zooms in on the clock. After a few seconds without any user input, the sketch falls into a 'screen saver mode', where the camera floats around.
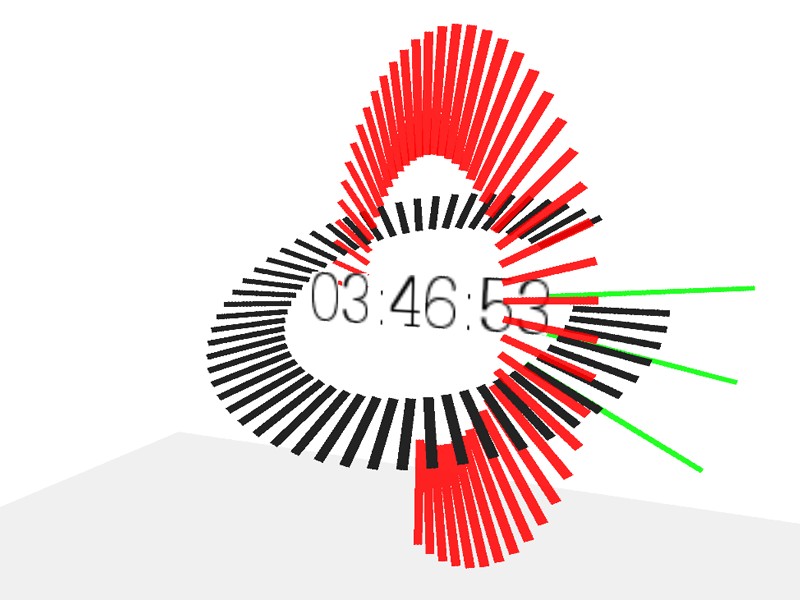
The Processing.js version sadly displays the time wrong on some browsers.
Run Dimension Clock with processing.js
Code: Dimension Clock
PFont font;
float timecount = 0;
float zoom=(200.0);
int mousemovefalse = 0;
int mousetimer = 0;
float mouseTestX = 0;
float mouseTestY = 0;
void setup()
{
size(800, 600, P3D);
frameRate(30);
background(255);
font = createFont("Helvetica", 24);
}
void draw()
{
//Screensaver-Modus
if (mouseX==mouseTestX&&mouseY==mouseTestY) {
mousetimer+=1;
} else {
mousetimer=0;
mousemovefalse=0;
}
if (mousetimer==120) {
timecount=0;
}
if (mousetimer>=120) {
mousemovefalse=100;
}
timecount+=1;
smooth();
background(255);
camera(mouseX+mousemovefalse*(sin(timecount/100)),
mouseY+mousemovefalse*(sin((timecount/100)*0.7)),
zoom, width/2.0, height/2.0, zoom-200, 0, 1, 0);
//Rasteranpassung für Darstellung
translate(width/2, height/2, -100);
//Boden
rotateX(-PI/2);
noStroke();
fill(240);
rectMode(CENTER);
translate(0, 0, 250);
rect(0, 0, 1000, 1000);
translate(0, 0, -250);
rotateX(PI/2);
//Zeit als Integers für Rechnung und Strings für Textdarstellung
String s = str(second());
int sec = second();
String m = str(minute());
int minu = minute();
String h = str(hour());
int hou = hour();
//Nullen für einziffrige Zahlen
if (sec<10) {
s = '0'+str(sec);
}
if (minu<10) {
m = '0'+str(minu);
}
if (hou<10) {
h = '0'+str(hou);
}
//Text
fill(0);
textAlign(CENTER, CENTER);
textFont(font);
text(h+":"+m+":"+s, 0, 0);
//Kreisförmige Anzeige für Sekunden
rotateX(PI/3.0);
for (int linesecond=0; linesecond<sec; linesecond++)
{
strokeWeight(4);
stroke(0, 220);
float winkels = radians(linesecond);
line(cos(winkels*6)*60,
sin(winkels*6)*60,
cos(winkels*6)*(200-2*sec),
sin(winkels*6)*(200-2*sec));
}
rotateX(PI/-3.0);
//Koordinatensystem wird zurückgedreht
//... Minuten
rotateY(PI/-4.0);
for (int lineminute=0; lineminute<minu; lineminute++)
{
strokeWeight(4);
stroke(255, 0, 0, 220);
float winkelm = radians(lineminute);
line(-cos(winkelm*6)*60,
-sin(winkelm*6)*60,
-cos(winkelm*6)*(200-2*minu),
-sin(winkelm*6)*(200-2*minu));
}
rotateY(PI/2.0);
//... Stunden
for (int linehour=0; linehour<hou; linehour++)
{
strokeWeight(4);
stroke(0, 255, 0, 220);
float winkelh = radians(linehour);
line(cos(winkelh*15)*60,
sin(winkelh*15)*60,
cos(winkelh*15)*(200-5*hou),
sin(winkelh*15)*(200-5*hou));
}
mouseTestX = mouseX;
mouseTestY = mouseY;
}
//Zoom per Mausklick
void mousePressed() {
zoom-=120;
}
void mouseReleased() {
zoom+=120;
}
Download Dimension Clock
Color Pattern
This sketch visualizes sine waves in different ways, based on time. There are two sine waves which are combined to produce a moving pattern by altering the vertex shapes. The colors of these shapes vary by the value of two sine waves with different frequencies. Altogether this sketch succeeds in creating an effect which can be compared to an optical illusion, while being based just on waves.
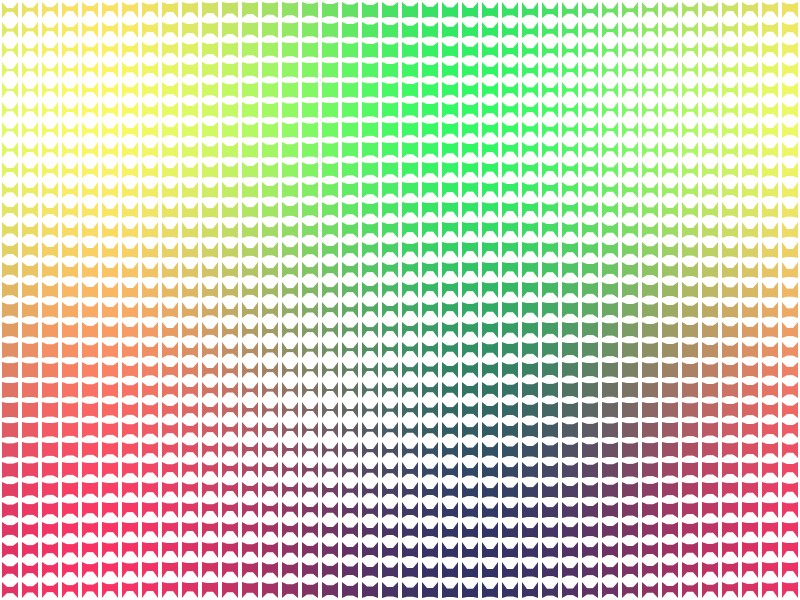
It may be a bit slow (depending on your system speed), but it works:
Run Color Pattern with processing.js
Code: Color Pattern
int col = 0;
int patternsize = 20;
void setup()
{
size(800,600);
background(255);
frameRate(30);
}
void draw()
{
background(255);
smooth();
col++;
for(int ex = 0; ex <= width; ex=ex+patternsize)
{
for(int yp = 0; yp <= height; yp=yp+patternsize)
{
float movex = ex;
float movey = yp;
noStroke();
fill(((sin(((movex/10)+col)/12))*100)+150,(sin(((movey/12)+col)/12)*100)+150,100);
beginShape();
float wob=col;
vertex( 2+movex, 2+movey);
vertex( 7+movex, (3*sin((20*wob+movey-movex)/100))+6+movey);
vertex(13+movex, (3*sin((20*wob+movey-movex)/100))+6+movey);
vertex(18+movex, 2+movey);
vertex(18+movex, 18+movey);
vertex(13+movex, (-3*sin((20*wob+movey+movex)/100))+14+movey);
vertex( 7+movex, (-3*sin((20*wob+movey+movex)/100))+14+movey);
vertex( 2+movex, 18+movey);
endShape(CLOSE);
}
}
}
Download Color Pattern
Spectralmandala
Another sketch based on sine waves. This sketch creates nine circulary arranged ellipses - floating around, changing their colors and shapes. The stretching and colorprogress suggest a three dimensional, nearly 'hallucinogenic' perception.
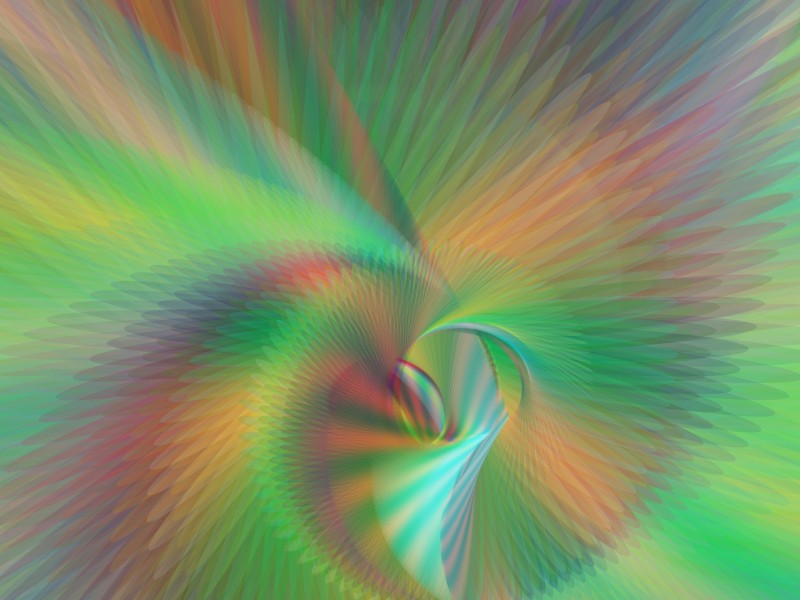
With Processing.js this sketch suffers from some mysterious interruptions. Apart from that it works fine.
Run Spectralmandala with processing.js
Code: Spectralmandala
float rad=radians(35);
float counter=0;
void setup()
{
size(800, 600);
frameRate(60);
background(255);
}
void draw()
{
counter=counter+0.01;
pushMatrix();
translate(width/2+sin(counter)*100,
height/2+sin(counter+PI/2)*100);
for (int i=0; i<10; i++)
{
noStroke();
rotate(sin(counter));
fill(122+122*sin(i-counter*counter),
122+122*sin(i+counter),
122+122*sin(i*i+counter), 30);
rectMode(CENTER);
ellipse(60, 60, 100*cos(counter*0.4),
100*tan(counter*0.6));
}
popMatrix();
}
Download Spectralmandala
Volumeter
The Volumeter uses the mic input to process speed, size and color of a moving circle. If the volume exceeds a specific treshold, the whole circle turns red and indicates that the sound is 'too loud'.
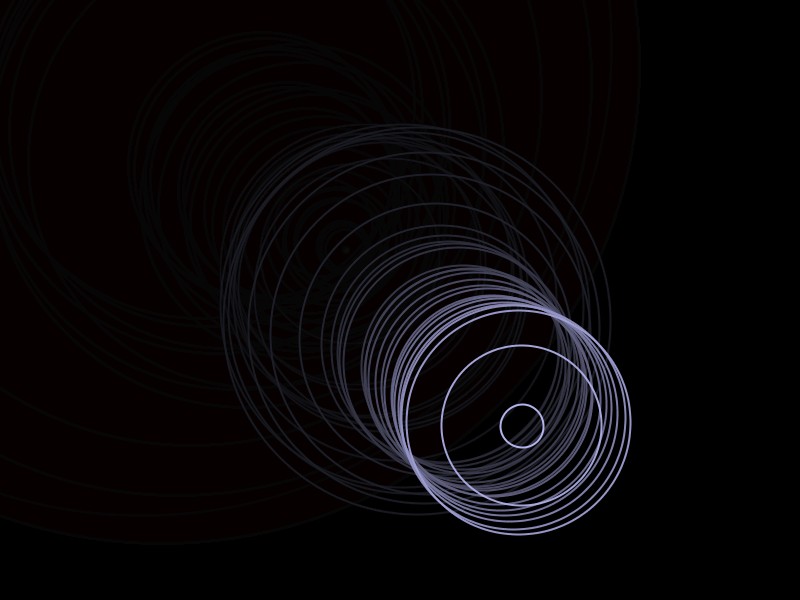
Code: Volumeter
import ddf.minim.*;
Minim minim;
AudioInput input;
float xmove=width/2;
float ymove=height/2;
int xaccel=1;
int yaccel=1;
float laut = 0;
void setup()
{
size(800, 600);
background(0);
minim = new Minim (this);
input = minim.getLineIn (Minim.STEREO, 512);
smooth();
}
void draw()
{
fill(0, 20);
noStroke();
rect(0, 0, width, height);
laut = input.mix.level()*4000;
noFill();
strokeWeight(2);
if (laut>=600) {
fill(255, 0, 0, 235);
}
xmove=xmove+laut*xaccel/50;
ymove=ymove+laut*yaccel/50;
if (xmove<=0) { xaccel=1; } if (xmove>=width) {
xaccel=-1;
}
if (ymove<=0) { yaccel=1; } if (ymove>=height) {
yaccel=-1;
} else {
stroke(195-laut/100, 195-laut/100, 255, 235);
}
ellipse(xmove, ymove, laut, laut);
}
Download Volumeter